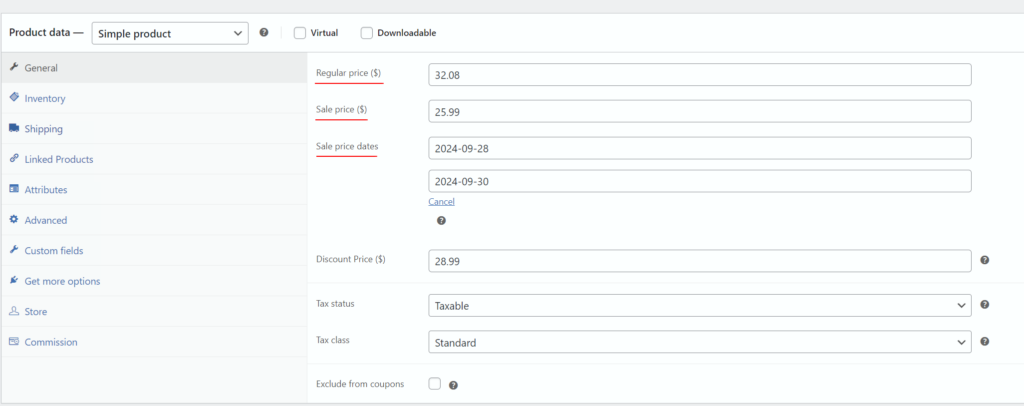
/* To add a Discount Price field in the WooCommerce product information, display it with the Regular Price when the sale price period ends, and ensure the product is sold at the Discount Price if it's entered */
// Add Discount Price Field to the General Tab in Product Data
function add_discount_price_field_to_product_data() {
global $post;
echo '<div class="options_group">';
// Discount Price Field
woocommerce_wp_text_input( array(
'id' => '_discount_price',
'label' => __( 'Discount Price ($)', 'woocommerce' ),
'placeholder' => __( 'Enter discount price', 'woocommerce' ),
'desc_tip' => 'true',
'type' => 'number',
'custom_attributes' => array(
'step' => '0.01', // Allow decimal numbers
'min' => '0'
),
'description' => __( 'This is the discount price for the product, applied when the sale period ends.', 'woocommerce' )
) );
echo '</div>';
}
add_action( 'woocommerce_product_options_pricing', 'add_discount_price_field_to_product_data' );
// Save Discount Price Field data
function save_discount_price_field( $post_id ) {
// Save Discount Price
$discount_price = isset( $_POST['_discount_price'] ) ? sanitize_text_field( $_POST['_discount_price'] ) : '';
update_post_meta( $post_id, '_discount_price', $discount_price );
}
add_action( 'woocommerce_process_product_meta', 'save_discount_price_field' );
// Display Discount Price when Sale period ends
function display_discount_price_if_sale_ends( $price, $product ) {
$regular_price = $product->get_regular_price();
$sale_price = $product->get_sale_price();
$discount_price = get_post_meta( $product->get_id(), '_discount_price', true );
$sale_end_date = $product->get_date_on_sale_to();
$current_date = new DateTime();
// Check if sale has ended and discount price is set
if ( ! empty( $discount_price ) && ( ! empty( $sale_end_date ) && $current_date > $sale_end_date ) ) {
// Display Regular Price with Discount Price
$price = '<del>' . wc_price( $regular_price ) . '</del> <ins>' . wc_price( $discount_price ) . '</ins>';
}
return $price;
}
add_filter( 'woocommerce_get_price_html', 'display_discount_price_if_sale_ends', 10, 2 );
// Apply Discount Price when Sale ends during Checkout
function apply_discount_price_on_checkout( $price, $product ) {
$discount_price = get_post_meta( $product->get_id(), '_discount_price', true );
$sale_end_date = $product->get_date_on_sale_to();
$current_date = new DateTime();
// Apply Discount Price if sale period is over and discount price is set
if ( ! empty( $discount_price ) && ( ! empty( $sale_end_date ) && $current_date > $sale_end_date ) ) {
$price = $discount_price;
}
return $price;
}
add_filter( 'woocommerce_product_get_price', 'apply_discount_price_on_checkout', 10, 2 );
add_filter( 'woocommerce_product_get_sale_price', 'apply_discount_price_on_checkout', 10, 2 );